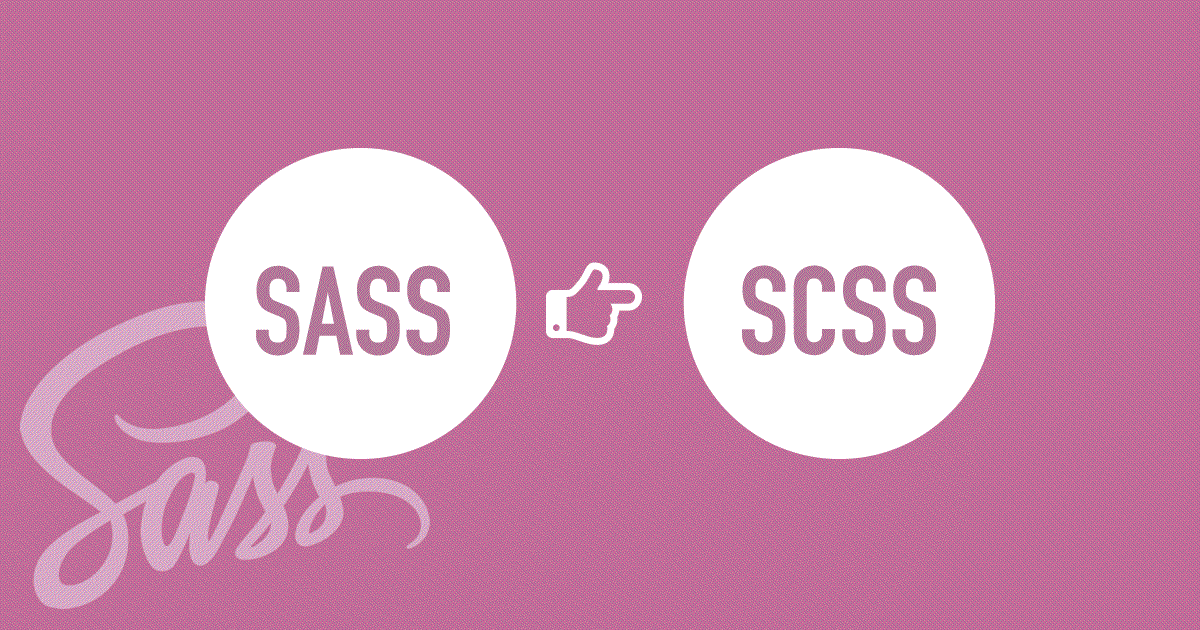
SCSS tips and tricks
Some things in CSS are a bit tedious to write, especially with CSS3 and the many vendor prefixes that exist. A mixin lets you make groups of CSS declarations that you want to reuse throughout your site. You can even pass in values to make your mixin more flexible. A good use of a mixin is for vendor prefixes. Here's an example for transform.
@function ConvertToREM($pxval) { $unit: unit($pxval); @if $unit == "px" { $val: parseInt($pxval); @return #{$val / $baseFontSize}rem; } @else { @return #{$pxval / $baseFontSize}rem; } } /*This mixin creates input Placeholder CSS. Example: */ /*@include placeholder { color: white; font-weight:100; }*/ @mixin placeholder { &::-webkit-input-placeholder {@content} &::-moz-placeholder {@content} &:-ms-input-placeholder {@content} &::placeholder {@content} } @mixin clearfix() { &:before, &:after { content: ""; display: table; clear: both; } } /*This duplicates Bootstrap 4's .container class.*/ @mixin container(){ width: 100%; padding-right: 15px; padding-left: 15px; margin-right: auto; margin-left: auto; @media (min-width: 576px) { max-width: 540px; } @media (min-width: 768px){ max-width: 720px; } @media (min-width: 992px) { max-width: 960px; } @media (min-width: 1200px){ max-width: 1140px; } } /*This mixin uses math to help calculate colors with opacity.*/ /*Use it as follows: background: alphaize(#73d6ff, #a0def5,0);*/ @function alphaize($desired-color, $background-color, $minimum-alpha: 0) { $r1: red($background-color); $g1: green($background-color); $b1: blue($background-color); $r2: red($desired-color); $g2: green($desired-color); $b2: blue($desired-color); $alpha: 0; $r: -1; $g: -1; $b: -1; @while $alpha < 1 and ($r < 0 or $g < 0 or $b < 0 or $r > 255 or $g > 255 or $b > 255 or $alpha < $minimum-alpha) { $alpha: $alpha + 1/256; $inv: 1 / $alpha; $r: $r2 * $inv + $r1 * (1 - $inv); $g: $g2 * $inv + $g1 * (1 - $inv); $b: $b2 * $inv + $b1 * (1 - $inv); } @return rgba($r, $g, $b, $alpha); }